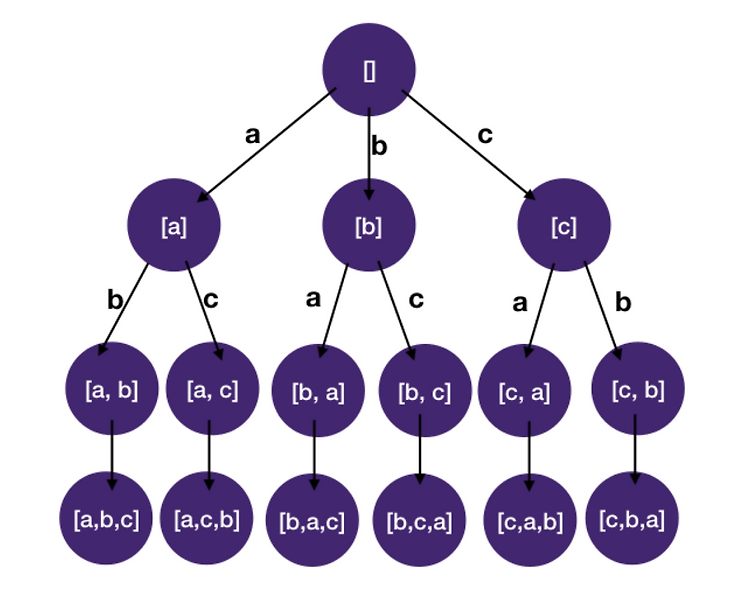
[LeetCode#46] Permutation
·
Algorithm/문제풀이
❒ Description제목Permutation링크https://leetcode.com/problems/permutations/description/자료구조비선형 (그래프)시간복잡도 O(n!)Permutation(순열)이란, 모든 가능한 경우를 그래프 형태로 나열한 결과라고 할 수 있다.이 문제는 BackTraking을 통해서 해결하는 문제인데, 모든 가능한 순열을 생성하기 때문에nums의 크기가 커질수록 시간이 급격히 증가한다. ❒ Solution1. Solution1public class Solution { public List> permute(int[] nums) { List> results = new ArrayList(); List elements = Arrays.s..